Setting up Recurring PayPal payments
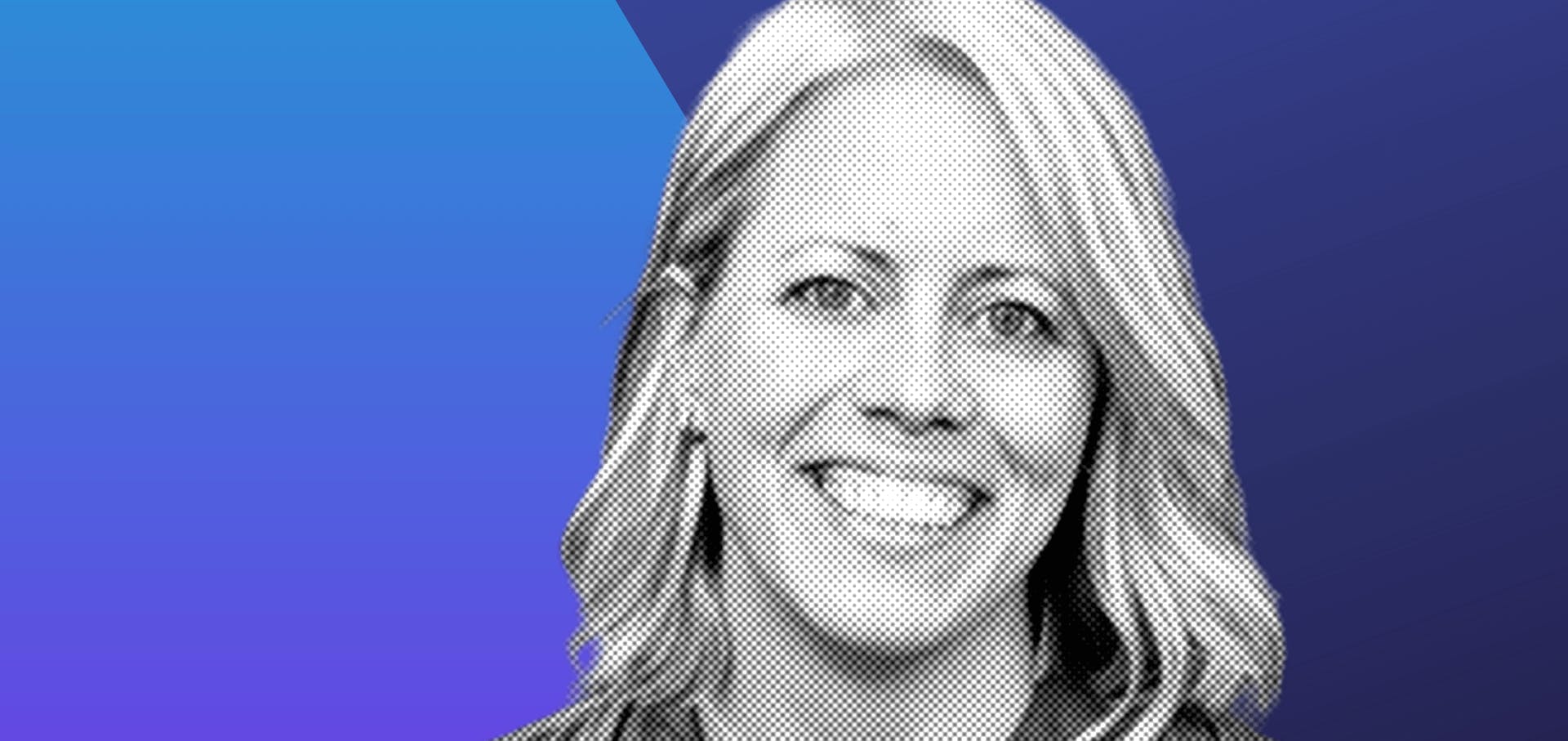
Nicki Lever
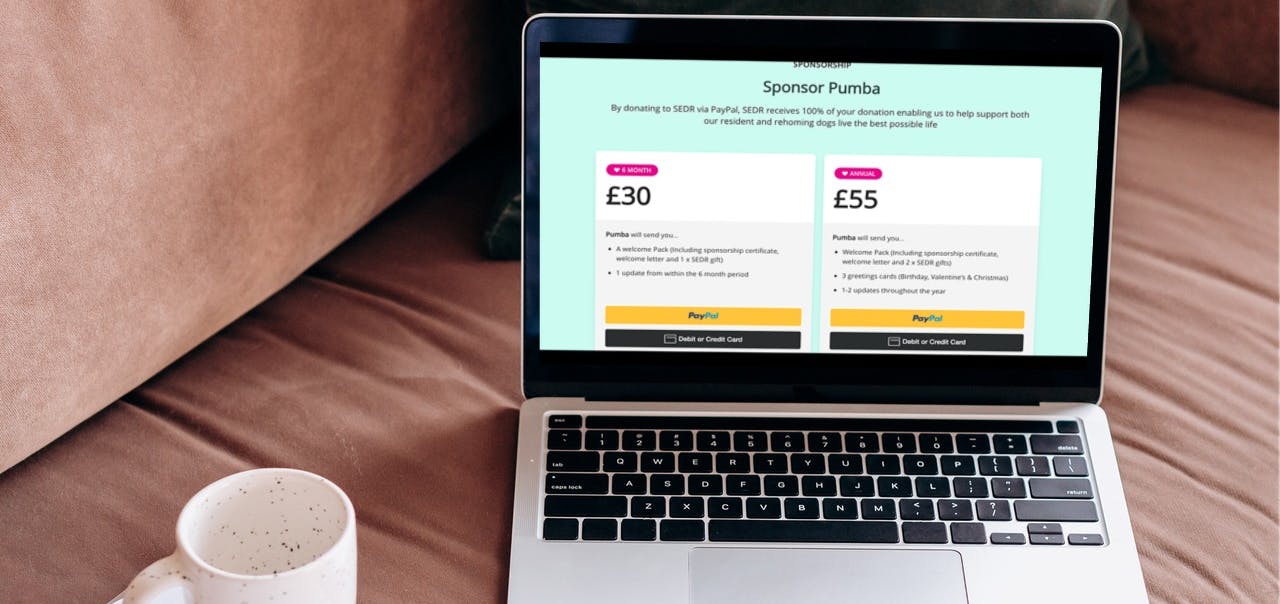
4 min read
If you build a lot of websites and you always use the same repository as a base and get to set up Netlify, how about using a script that just asks for the name of your new repository and gets the new website up and running?
I will go through the process of building this script and the issues encountered.
You will need to have
From Github
From Netlify
First, we want to create our new repository in Github from a template repository, well their API will do that for you
const repoInfo = await octokit.repos.createUsingTemplate({ template_owner: config.githubOrganization, template_repo: githubTemplateRepo, owner: config.githubOrganization, name: projectName, include_all_branches: false, private: true })
That’s it, now we have a new repository in Github from our template repository.
The Github repository is set up, now we create a website on Netlify using their API too:
return client.createSite({ body: { name: domain, repo: { cmd: 'gatsby build', dir: 'public', id: githubRepoId, provider: 'github', repo_type: 'git', repo_url: `https://github.com/${config.githubOrganization}/${projectName}`, repo_branch: config.githubBranch, repo_path: `${config.githubOrganization}/${projectName}`, deploy_key_id: deployKey } } })
Well, this is ok but it won’t be able to build the website because it may not have enough permissions.
To fix this, We are going to link the Github repository to Netlify before that we create it in Netlify.
So before we create the website in Netlify we need to get a few things:
To do this, we need to get the Github repository id, well we are in luck, when we created the repository, using the Github API, it returns the id.
Now the Netlify deploy key and its id, for this we will use the Netlify API:
return client.createDeployKey()
After we need to send the deploy key to Github, using the Github API:
octokit.repos.createDeployKey({ owner: `[githubOrganizationOrUserId]`, repo: `[newProjectName]`, key: netlifyDeployPublicKey })
We have basically linked Github and Netlify. Now we can create our website on Netlify just by calling the same function as before but by adding the Github repository id and Netlify deploy key:
return client.createSite({ body: { name: `[netlifySubdomain]`, repo: { id: githubRepoId, provider: 'github', repo_type: 'git', repo_url: `https://github.com/${githubOrganizationOrUserid}/${newProjectName}`, repo_branch: ‘main’, repo_path: `${githubOrganizationOrUserid}/${newProjectName}`, deploy_key_id: deployKey } } })
You have, now, a website up and running in Netlify with a subdomain that you have chosen.
If you are using Gatsby to build your website in Netlify you need to add the command of how to build it and its path.
To do this, you will need to modify the Netlify create site:
return client.createSite({ body: { name: `[netlifySubdomain]`, repo: { cmd: 'gatsby build', dir: 'public', id: `[netlifySubdomain]`, provider: 'github', repo_type: 'git', repo_url: `https://github.com/${githubOrganizationOrUserid}/${newProjectName}`, repo_branch: ‘main’, repo_path: `${githubOrganizationOrUserid}/${newProjectName}`, deploy_key_id: deployKey } } })
Now that your script is working, you could wrap this in:
This should help you create and deploy websites quicker without doing all the manual process for the setup, no one loves repetitive processes.
From this, you could add the extra processes that you need for the creation of your website.
You can see the full code here.
References
As with everything in life, things pan out better when you work together. So if you are looking for a website support, updating a Shopify Theme, a fresh out of the box site or support with your Digital Strategy, you've come to the right place. We pride ourselves on our flexibility and honesty.
Why not get in touch?